Working with Recyclerview and Cardview
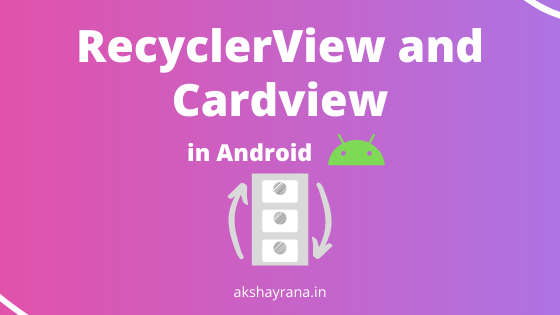
Hello World, Today we are going to see how we can make a simple recyclerview with cardview in android studio. In this article, you will learn how to work with recyclerview, what is recyclerview layoutmanager? See the below gif for understanding what we are going to build.
Let's start...
What is Recyclerview in Android?
Recyclerview is an advanced version of the listview. It is used to make the repeated view and recycle them to save more memory. Recyclerview is way more efficient than listview. Recyclerview easily handles a large set of views and is very flexible. Recyclerview is like a container that renders a large set of views very efficient manner. Recyclerview is optimized to work with a large number of views and save lots of memory so that your app will freeze or hang the device.
According to the
official android site:
The RecyclerView widget is a more advanced and flexible version of
ListView.
In the RecyclerView model, several different components work together to display your data. The overall container for your user interface is a
RecyclerView object that you add to your layout. The RecyclerView fills itself with views provided by a layout manager that you provide. You can
use one of our standard layout managers (such as LinearLayoutManager or
GridLayoutManager), or implement your own.
What is Cardview in Android?
CardView
is a widget provided by the android to build a new look and efficient UI. You can make your app look more professional see examples below.
Cardview
is an amazing concept which makes your user experience better than old android
UI. Cardview was released with Android version 5.0 which is android lollipop.
I have already explained
what is cardview, you can read about it
here.
Enough talking now let's go to the coding part. Yay!
Using Cardview in RecyclerView with example
Making recyclerview with cardview is very simple. We need to follow certain
steps which are listed below.
Step 1. Add required Dependencies in your project.
We use below dependency in our project to use recyclerview and cardview.
As we are
loading images from the internet
we also use
glide dependency
in our project, if you want to learn how to use glide then you can read
this article.
Step 2. Make Data Model Class for Holding Data
First, we need to make a way to hold data that we are going to use populating
in the recyclerview items.
Make a new java file in the package and we name it as ProfileModel.java.
And in this file, we add below code:
In the above code, we have a class name ProfileModel which has a private string
variables firstName, lastName, photo, bottomColor.
After that, we have a construct with all the variables as parameters to
initialize our field variables.
And then, we make the getter method for each and all the variables to get its
values.
Simple and straight forward.
Step 3. Make an item layout file for recyclerview.
In this step, we will make a layout for our a single row of recyclerview so
that recyclerview uses this layout and make copies of items and add data in
the layout if you are unable to understand what I said then don't worry you
will understand later.
To make the item layout click on the layout folder under res folder in your project and click on new and then click on the layout resource file. We will name this as profile_item and hit enter.
See below, what our main components for our item layout.
We have a cardview as the main container and an imageview that shows the profile picture and a textview to display the name of the person and in the
bottom a solid color border to make a decoration in the cardview.
To make this layout we are using the below code.
We specific the height of the cardview and make rounded border any using
cardCornerRadius attribute.
To use multiple views in the cardview we need to have a container layout in
the cardview.
We can't use cardview as a container we must add a layout for multiple views
as child.
Next is, to make image round or circular we can use cardview and take advantage of cardCornerRadius attribute. Have you noticed in this cardview we are not using and container because we are not using multiple views we are using only ImageView as a child so no need to add any container.
See also:
Save Image to storage using Glide
After that, we added a textview to display name and in the last add a
view for showing a border as decoration.
We have also set the id to all the views for later use.
Step 4. Make the Adapter class for recyclerview.
In this step, we will make an adapter class that will help to populate the
data in the view and make item layout copies in the recyclerview.
Let's start by making a new java file and name it as ProfileAdapter.
After that, we need to extend this class with RecyclerView.Adapter.
At this point, you will see the error in you code. Don't worry and keep going
with the article.
Now, we need to create another class for view holder but this one is going to
be an inner class of the ProfileAdapter class and this class will extends
RecyclerView.ViewHolder.
At this point, you will see an error in the ProfileViewHolder, to resolve this error you need to make a default constructor with the View parameter and call the super constructor. See the below code.
Now in the ProfileViewHolder class, we will make declare all the view which we want to populate the data. So we declare an ImageView for the photo, a
TextView for user name, and View for bottom line background color.
After that, we will initialize them in the contractor with their corresponding ids using the itemView variable from constructor parameter. See the below code.
Now come to adapter class, we made our viewholder class now its time to make
use of it in the adapter class as generic as below.
Now we need to implement the required methods these methods are as follows:
- onCreateViewHolder
- onBindViewHolder
- getItemCount
These are the mandatory methods of a Recyclerview adapter.
Implement them like below.
We need to make a constructor for our adapter class so that we can pass data
from our activity to adapter class.
Make a constructor like below.
In the above code, we are getting context and data as in the list and save the
values to the global variables.
Now come to the methods, let's start with the third method getItemCount.
in this method, you have to return the number of items you want to make in the
recyclerview.
So we simply return our data's length or size. in this case, we use the size
method of the list as we will get our data in the list.
Now come to the first method, this method class for the first time when we pass an adapter to recyclerview. In this method, we will inflate our item layout which we previously made. See the code for the reference.
And this comes the last method, onBindViewHolder method is called when view binds with the recyclerview and in this method, we write our logic for populating the data in the view. see below for better understanding.
and this ends the adapter part and probably the longest one.
The whole code should look like this.
Step 5. Set Layout Manager and Adapter to RecyclerView.
Now we can set layout manager and adapter but before doing this we need to prepare our list of data and initialize recyclerview after that we can set all the things. See the code below.
and that's it now run your app and take a look at amazing recyclerview with
cardview.
If you like this article, do share this article with your batchmates and friends.
And also you can subscribe to our newsletter.
Share this Article 👇
Subscribe to our Newsletter
By subscribing to our newsletter you're agreeing with our T&C.